Contents
from PIL import Image, ImageDraw, ImageFont
!ls /usr/share/fonts/truetype/dejavu/
DejaVuSans-Bold.ttf DejaVuSansMono.ttf DejaVuSerif-Bold.ttf
DejaVuSansMono-Bold.ttf DejaVuSans.ttf DejaVuSerif.ttf
im = Image.open('SS9_car_305_top_40.png')
position = (100, 100)
text = "Explanatory text"
font = ImageFont.truetype('/usr/share/fonts/truetype/dejavu/DejaVuSerif-Bold.ttf', 64)
color = (0, 0, 0)
# get text size
text_size = font.getsize(text)
# initialise the drawing context with the image object as background
draw = ImageDraw.Draw(im)
draw.rectangle([position, (position[0]+text_size[0]+10,position[1]+text_size[1]+10)], fill='orange', outline='orange', width=5)
draw.text(position, text, fill=color, font=font)
draw.line([(0,0),position], fill='red', width=5)
im
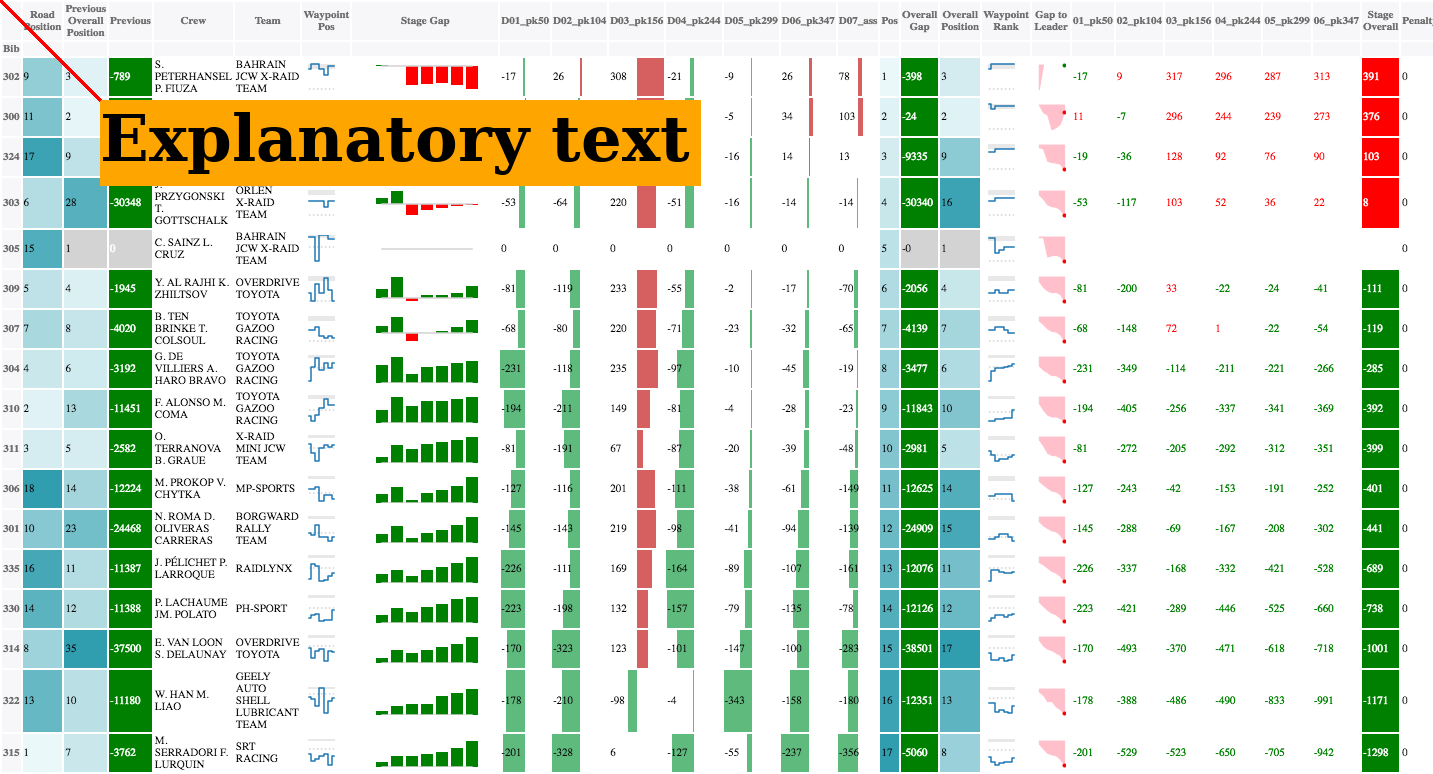
The following will load in the matplotlib interactive backend, then when you hove the cursor over the image, it will show the current co-ordinates.
%matplotlib notebook
import matplotlib.pyplot as plt
plt.imshow(im)
<matplotlib.image.AxesImage at 0x7f02ae8e39b0>
#https://stackoverflow.com/a/52574134/454773
coords = None
%matplotlib notebook
import matplotlib.pyplot as plt
import numpy as np
import ipywidgets as wdg # Using the ipython notebook widgets
fig = plt.figure()
plt.imshow(im)
# Create and display textarea widget
txt = wdg.Textarea(
value='',
placeholder='',
description='Co-ords:',
disabled=False
)
display(txt)
# Define a callback function that will update the textarea
def onclick(event):
global coords
coords=event
txt.value = f'{coords.x}, {coords.y}' # Dynamically update the text box above
# Create an hard reference to the callback not to be cleared by the garbage collector
ka = fig.canvas.mpl_connect('button_press_event', onclick)
coords.x, coords.y
(500, 363, 491.145759828629)
dir(coords)
['__class__',
'__delattr__',
'__dict__',
'__dir__',
'__doc__',
'__eq__',
'__format__',
'__ge__',
'__getattribute__',
'__gt__',
'__hash__',
'__init__',
'__init_subclass__',
'__le__',
'__lt__',
'__module__',
'__ne__',
'__new__',
'__reduce__',
'__reduce_ex__',
'__repr__',
'__setattr__',
'__sizeof__',
'__str__',
'__subclasshook__',
'__weakref__',
'_update_enter_leave',
'button',
'canvas',
'dblclick',
'guiEvent',
'inaxes',
'key',
'lastevent',
'name',
'step',
'x',
'xdata',
'y',
'ydata']
#im.show()
image_name_output = '03_add_text_to_image_02_input_01.jpg'
im.save(image_path_output + image_name_output)
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-4-d8b9363dcf66> in <module>
6
7 image_name_output = '03_add_text_to_image_02_input_01.jpg'
----> 8 im.save(image_path_output + image_name_output)
NameError: name 'image_path_output' is not defined
%matplotlib inline